Jul. 15, 2013
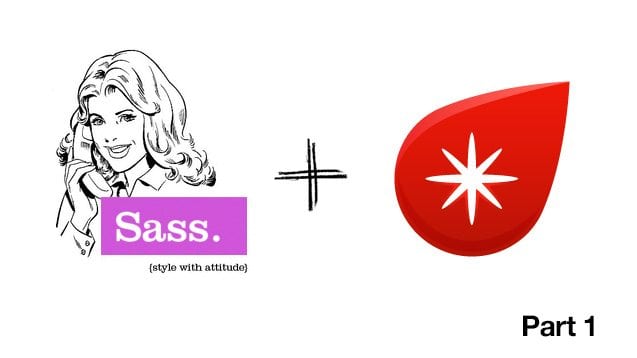
Getting started with Sass (SCSS) and Compass Part 1
What is Sass (SCSS)?
For those of you who haven’t yet got their feet wet in the world of CSS preprocessors, I’m going to quickly explain what they do. CSS Preprocessors allow you to rapidly code modular CSS and super clean markup with features such as variables, mixins, nested selectors and more. Don’t worry, I will discuss all of these concepts in more detail later on.
The two most popular CSS preprocessors in use on the web today are Less.css and Sass, which is arguably the more powerful of the two thanks to the compass framework. If you’ve visited the Sass Website you may have noticed that there are two different versions of the syntax. I’m going to show you how to use Sass with the SCSS syntax which is closely related to the CSS3 you already know and love.
Let’s get started
How do I use SCSS in my stylesheets? While everything can be done via the command line (Sass compiles the fancy CSS documents using Ruby), the easiest way (and my recommended method) is to use one of the existing tools that does all that complicated command line stuff for you. My tool of choice for the Mac is Codekit which I highly recommend and is easily worth the $25. It also comes with a free trial so you should download it like, now. For those of you on Windows, I would recommend using Prepros (also available for Mac, and completely free)
The codekit website has some tutorials on how to get started and the prepros homepage has a comment section with some tips on how to get set up. There are also other resources available such as this codekit tutorial here.
Where does Compass fit in with all this?
I want to jump right into Compass as it is probably the #1 reason why most people make the jump from CSS to Sass. Compass is a CSS framework that provides some awesome functionality out of the box for Sass. It makes use of several more complex features in Sass however it is very easy to use without fully understanding those features. If you’re not using an app like Codekit or Prepros, you’ll need to make sure you install Compass via the command line before proceeding.
For the sake of keeping today’s post simple, I’m going to look at an example from the CSS3 module, so let’s go ahead and import that at the top of our document.
@import "compass/css3"
Great, now I’m going to show you how you can forget about memorizing browser prefixes. Yup, awesome right? One of the most painful parts of coding CSS these days as a developer is remembering all those pesky browser prefixes which are critical for providing cross-browser and backward compatibility.
Let’s use border-radius (view compass docs) as an example. We’re going to keep it simple, but you’re allowed to use all the wild shorthand craziness that you like.
Regular CSS
.button { -webkit-border-radius: 3px; -moz-border-radius: 3px; -khtml-border-radius: 3px; border-radius: 3px; }
SCSS
.button { @include border-radius(3px); }
Where have you been all my life? Those were my thoughts when I first saw this feature in action. Compass has a whole slew of these CSS3 helpers.
Let’s look at another commonly used Compass mixin, box-shadow. You’ve most likely been through the pain before of prefixing all your box-shadow statements.
Regular CSS
#box-shadow { -webkit-box-shadow: 0px 0px 5px #000; -moz-box-shadow: 0px 0px 5px #000; box-shadow: 0px 0px 5px #000; }
SCSS
#box-shadow { @include box-shadow(0px 0px 5px #000); }
You should have the general idea of how these work by now. For a full list of Compass, CSS3 mixins click here. I’ll cover some more complicated examples in a later post.
Nesting with SCSS
I want to talk about nesting now as it’s one of the most useful and potentially damaging functions in the Sass toolkit. Potentially damaging? Why? Because it becomes extremely tempting to write beautiful, organized CSS. Wait, what’s wrong with that? The problem is that the more beautiful your nesting looks, the more bloat you’ve just added to your code via long selectors. Long selectors should be avoided like the plague for reasons such as efficiency, dependency, and specificity, however, sometimes you will want to select a couple of levels down and that’s where nesting should be used. Let’s take a look at an example of nesting in SCSS before we move on any further.
Let’s assume in these examples that #header-nav is a UL element. This is a perfect use-case for nesting; it lets us organize our code, write code quicker and make it more readable. At the same time, it doesn’t abuse the nesting feature to generate badly optimized selectors.
Regular CSS
#header-nav { list-style-type: none; } #header-nav li { display: inline-block; *display: inline; zoom: 1; margin-left: 15px; } #header-nav li:first-child { margin-left: 0; } #header-nav a { text-decoration: none; color: #3f3f3f; } #header-nav a:hover { color: #000; }
SCSS
#header-nav { list-style-type: none; li { @include inline-block(); margin-left: 15px; &:first-child { margin-left: 0; } } a { text-decoration: none; color: #3f3f3f; &:hover { color: #000; } } }
The first thing you’ll notice is that the SCSS has #header-nav written once versus 5 times in the regular CSS example; this is the beauty of nesting. It also provides improvements to readability and maintainability when the element grows in complexity. I’ve used several features of nesting here and I’ll explain what each one does.
Looking at the li selector, you can see that it’s a ‘second level’ selector underneath the #header-nav selector. When SASS compiles this, the li selector effectively becomes #header-nav li. Going deeper into the li declaration I have another nested selector, &:first-child. This in turn results in #header-nav li:first-child post-compilation. There are other uses for nesting using & , which I’ll cover in greater detail another day.
I’m going to wrap up here for today, but next week I’ll introduce variables and some more complicated SCSS techniques so stay tuned.
If you have any questions feel free to leave a comment!
Book with us
Let’s accomplish what you are looking for, our team of experts are here for you.
Let's work togetherWarning: Working with our team may result in excessive creativity, uncontrollable 'aha' moments, and an addiction to perfect pixels. Please proceed with caution.